Consuming the Live Currency Conversion Web Service in WPF VB.NET
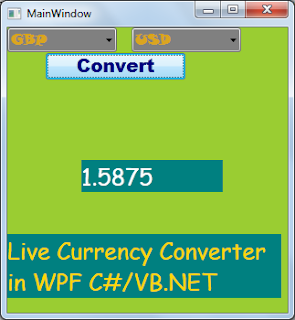
Step 1) Add Currency/Exchange convertor WebService Service reference into WPF Application.
Here I choose http://www.webservicex.net/CurrencyConvertor.asmx?WSDL Webservice
Step)Add 2 combo boxes and 1 button control and one TextBlock to XAML(as shown below)
<ComboBox HorizontalAlignment="Left" VerticalAlignment="Top"
Width="120" Grid.Row="0" x:Name="cbxfrom" Tag="from"
Foreground="Goldenrod" Background="gray" FontFamily="Snap ITC" FontSize="16"
/>
<ComboBox HorizontalAlignment="Left" VerticalAlignment="Top"
Width="120" Grid.Row="0" Margin="136,0,0,0"
x:Name="cbxto" Tag="to"
Foreground="Goldenrod" Background="gray" FontFamily="Snap ITC" FontSize="16"
/>
<Button Content="Convert" HorizontalAlignment="Left"
VerticalAlignment="Top"
Width="153" Grid.Row="0" Margin="42,28,0,0"
Click="Button_Click_1"
Foreground="Navy" Background="BlanchedAlmond" FontFamily="Arial Black" FontSize="20" FontStyle="Italic"
/>
<TextBlock HorizontalAlignment="Left" TextWrapping="Wrap" Text="TextBlock"
VerticalAlignment="Top"
Grid.Row="1" Margin="81,85,0,0" Width="155"
FontFamily="Comic Sans MS" FontSize="25" Foreground="white" Background="teal"
x:Name="txtConvValue"
/>
Step 3) In the Convert Button Event handler
Calling web Service Method
Private Sub Button_Click_Converter(sender As Object, e As RoutedEventArgs)
Dim from As [String] = TryCast(cbxfrom.SelectedValue, [String])
Dim [to] As [String] = TryCast(cbxto.SelectedValue, [String])
Dim convValue As Double = client.ConversionRate(DirectCast([Enum].Parse(GetType(CurService.Currency), from), CurService.Currency), DirectCast([Enum].Parse(GetType(CurService.Currency), [to]), CurService.Currency))
txtConvValue.Text = convValue.ToString()
End Sub
Step 4) Here Currency converter Namespace is CurService
Dim client As CurService.CurrencyConvertorSoapClient = New CurrencyConvertorSoapClient()
Step 5) Load Currency Codes to Combobox
Private Sub LoadCurrencyCodes()
Try
Dim CurCodes As [String]() = [Enum].GetNames(GetType(CurService.Currency))
cbxto.ItemsSource = CurCodes
cbxfrom.ItemsSource = CurCodes
Catch ex As Exception
MessageBox.Show(ex.Message)
End Try
End Sub
Here is the Output